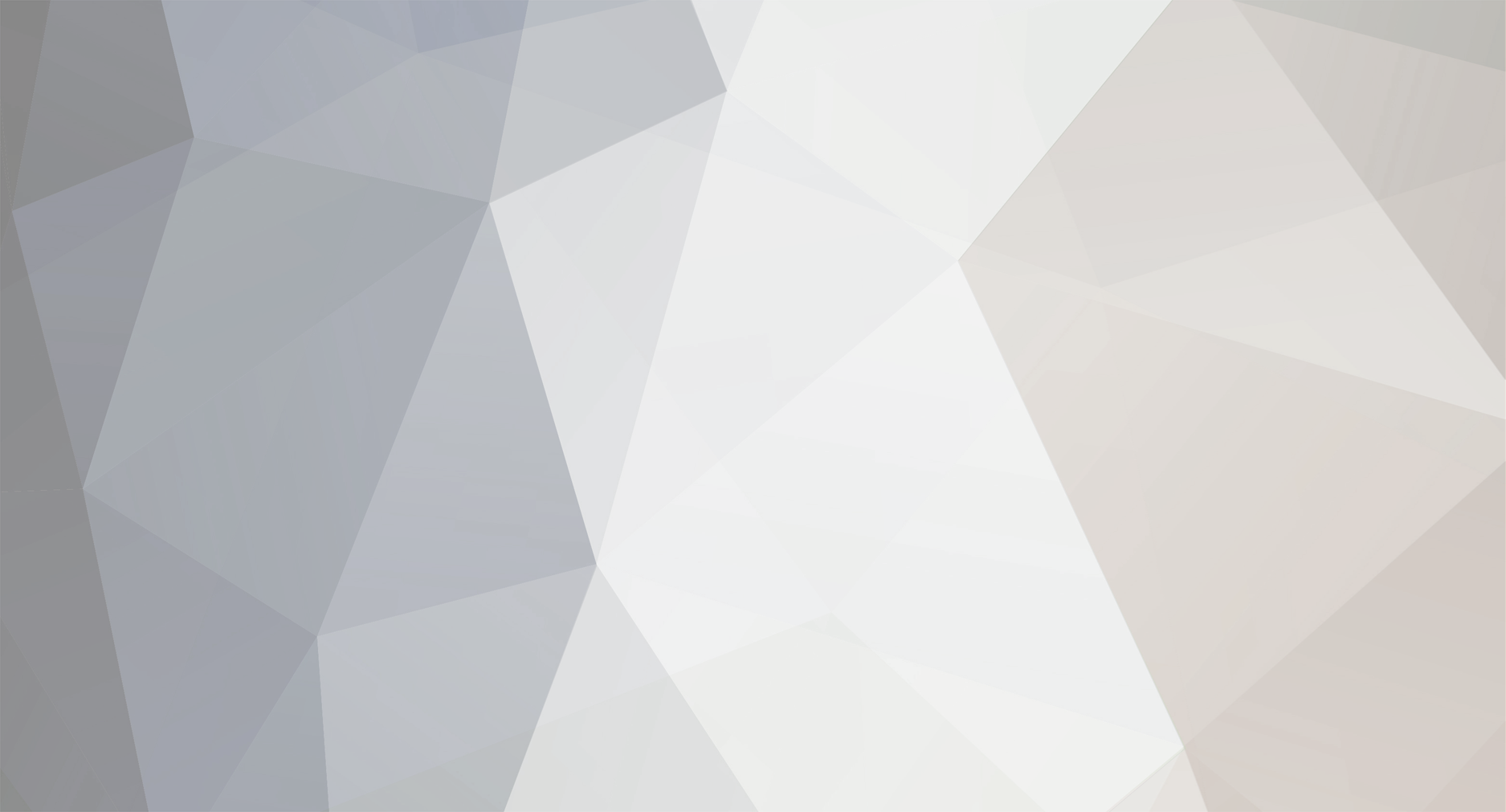
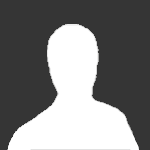
Humble_Bee
Members-
Posts
112 -
Joined
-
Last visited
Content Type
Profiles
Forums
Downloads
Jobs Available
Server Database
Third-Party Services
Top Guides
Store
Everything posted by Humble_Bee
-
I know that pc_checkskill works on players to find out what level of a skill they have learned (if at all), and I'm looking for a similar function for mobs. If one doesn't exist, does anyone know the path the src would check? Is it something like "mob_skill[skill_id].skill_lv"? I tried coding: int skill_checklevel(struct block_list *src, uint16 skill_id) { if (src->type == BL_MOB){ struct mob_data *md = (struct mob_data*)src; struct mob_skill *ms; int i; int level = 0; nullpo_ret(ms = md->db->skill); for (i = 0; i < MAX_MOBSKILL; i++){ if(ms[i].skill_id == skill_id){ level = ms[i].skill_lv; break; } else{ level = 0; } } return level; } } And it didn't work. I've also tried a few other variations, and they didn't work either. If I knew the path the src followed, I could probably figure it out, but I haven't yet. I want to be able to make passive boosts for mobs based on skill level known, but have been stumped.
-
Does anyone have the bug where pets won't attack the same mob type they are? Like if I have a poring pet, it won't attack porings. Same goes for all pets not attacking monsters of their breed. Does anyone know where this is in the source code? I'm not sure if it's based in the mob code, the pet code, or elsewhere, but it's annoying.
-
Trying to figure out how to make it so that all pets would get a skill. I know I could add it one by one, but that would be a pain.
-
Right now, in the game, it looks like there aren't any non-ground moves that have a target cap. Anyone have any ideas on where to start to make a setting for target caps for skills?
-
I'm trying to make all my stats balanced, and this is pretty easy with ideas like accuracy, damage, and damage resist, but I'm having a hell of a time with regen. While accuracy, damage, and damage resistance all interact with each other, regen does not nicely interact with them. If 1 SP allows you to do 10 damage, and you start with 120 SP, then you could do 1200 damage before you run out. If each attack takes 1 sec to cast, it would take you 120 secs to run out of SP, and then you would have to wait to until you regenerated your SP. If you regen 3% Base SP per 6 seconds (not adding bonus Max SP values in), it would take you 240 seconds to get to full. Now, if I put this into total damage output over time, this base would look like this: At 2 minutes (No Stat Boosts): 60 SP Leftover 1200 damage done At 16 minutes (No Stat Boosts): 0 SP leftover 6000 damage done And then if you compare a double regen boost vs a double Max SP boost, it would look like this: At 2 minutes (Regen Doubled): 120 SP leftover 1200 damage done At 16 minutes (Regen Doubled): 120 SP leftover 9600 damage done -- At 2 minutes (Max SP Doubled): 120 SP leftover 1200 damage done At 16 minutes (Max SP Doubled): 0 SP leftover 7200 damage done As you can see, in the long run, depending on how you set base regen, when you double it, it doesn't tend to balance out. This is even more problematic for HP regen, because if you double 3% HP per 6 seconds, it becomes 1% HP per second, which usually is more than most people think is balanced. On top of this, we come into the problem of potions. If a game is using potions or Inns, a person then has to calculate the value of regen vs how cheap potions are or how time consuming it is to get to an inn. If potions are cheap, regen loses value. If it's easy to travel and get back to an inn, regen also loses value. It can be a really tough concept to balance with stat boosts. Any ideas on how you all would balance it?
-
Adding amotion delay to monster's attacks after player missed attack
Humble_Bee posted a question in Source Support
So I've gotten monsters to attack players on missed attacks by coding: // check if we're landing a hit if(!is_attack_hitting(&wd, src, target, skill_id, skill_lv, true)){ wd.dmg_lv = ATK_FLEE; if (target->type == BL_MOB){ struct mob_data *md = (struct mob_data *)target; if (md->attacked_id != src->id){ sc_start4(target,target, SC_MODECHANGE, 100, 1, 0, MD_AGGRESSIVE, 0, wd.amotion); }; } } But my problem is- there is no amotion delay. A player might not even finish firing an arrow and a monster is already on top of him. Is there any easy way to make the mode change happen after the weapon animation has happened? -
[Showcase] Upscaled NPC sprites
Humble_Bee replied to Balfear's topic in Spriting & Palette Showcase
You repacked jpegs or whatever format the pics were in back into sprites one by one? If so, ouch. That's like licking 17,000 stamps for envelopes. -
[Showcase] Upscaled NPC sprites
Humble_Bee replied to Balfear's topic in Spriting & Palette Showcase
So the neural network was able to apply a formula, so to speak, to all the sprites quickly? They are definitely sharper. I will say, Gibbit looks like it is hanging Luchadores when in high-def. heh. -
When doing double attack while dual-wielding, the game always reverts to using the main weapon (R Hand) for the final hit. Does anyone know where this logic is in the code? I've set my dual-wield double attacks to hit 4 times, but the game does three hits of the main weapon and one hit of the L-Hand weapon. I'd like the game to do two hits of each. Ideas?
-
I see the original coders initialize bl and src all the time and their code works fine, but I can't figure out how to initialize them myself. How do I add bl or src info into the middle of a function without the game spazzing? I have this much accomplished: struct block_list *bl; ??? status_get_level(bl) I just don't know what bl equals so that it will retrieve the data appropriately. Thanks for any help!
-
How risky is it using RO sprites in another game?
Humble_Bee replied to robby22's topic in Off Topic
Can you get sued? Yes. Will you? That's a roll of the dice. Imagine being one of the graphic designers who spent hours carefully filling in all those pixels. You busted your rear end just to build one character, not even including weapons and armor, and then someone just rips it and tosses it in their video game and doesn't give you a dime or even a thank you. Would you be upset? Something to keep in mind. That's why I hope the video game community pushes for a public domain library of assets (maps, monsters, icons, etc), because in the long run, it could help a lot of enjoyable games to come out. -
Note: I know this question is old, but I don't see the answer on the boards, and my version of rAthena doesn't have a quick solution, so I made one, and I imagine it will help people. You can go into mob.cpp and find the section that talks about mob drops and add in slave mobs as a section there. Mine looked like this: if( !(type&1) && !map_getmapflag(m, MF_NOMOBLOOT) && !md->state.rebirth && ( !md->special_state.ai || //Non special mob battle_config.alchemist_summon_reward == 2 || //All summoned give drops (md->special_state.ai==AI_SPHERE && battle_config.alchemist_summon_reward == 1) //Marine Sphere Drops items. ) ) And I changed the top line to look like this: if( !(type&1) && !map_getmapflag(m, MF_NOMOBLOOT) && !md->state.rebirth && !md->master_id && ( My slave mobs are no longer giving drops.
-
This is my code for a race changing AT Command (the very basic start of one- I'll add more later). It is not currently updating the player's race though. Can someone more familiar with C++ show me what I would need to change? I know that stat updates show up in like 5+ places in rAthena code, so I'm not sure how much more work I'll need to do it. You would think it would be as easy as querying the server for the data and using some sort of set command, but I only "talk" the vocabulary, I don't actually know how to use it. Ha. ACMD_FUNC(changerace) { char xrace[20]; struct status_data *base_status; base_status = &sd->base_status; nullpo_retr(-1, sd); memset(atcmd_output, '\0', sizeof(atcmd_output)); if (sscanf(message, "%20s",&xrace) > 0) { if (xrace == "Formless" ) sd->base_status.race = RC_FORMLESS; } return 0; }
-
Is there code in the source that checks what max hp is supposed to be at lv. 1, so that it can set actual hp to match max hp? I turned off the job_basehpsp_db.txt file option so I could use a formula (pc_calc_basehp, but I modified it), and my characters were starting not with max_hp, but a much lower hp amount. I found out how to temporarily fix it by going into char.cpp, as far as making the actual hp match my max hp for a starting novice, but it seems like it would be a lot easier just to have a formula decide all this -> i.e.- have pc_calc_basehp (in pc.cpp) get read, and then have actual hp be set to match that. This way char.cpp wouldn't need to be adjusted all the time. My current fix doesn't take care of dorams if I wanted them to have a different hp amount, and the same goes for other races when I add them to the game in the future. Maybe I'm missing something? Note: I'm not asking for support. I'm assuming my problem is actually a current design feature when not using job_basehpsp_db.txt, and so I'm discussing the suggestion of inserting a formula/function that reads max_hp and matches actual hp to it on character creation. (Though my version of rAthena is quite a bit older, so perhaps this has been fixed already *blush*)
-
Any general ideas why a character's HP wouldn't equal it's Max HP on character creation? This might be related to the fact that I went with a formula in base_hp instead of using the job_basehpsp_db.txt file (there is an option to disable it and use a base formula), but I can't figure out why the hp still wouldn't updated to match the max_hp on character creation. Is there a way to fix the issue with a status update force if people can't guess what is wrong? EDIT: A bit of a headache, but apparently rAthena doesn't connect base_hp (max) with actual hp on character creation by default. I had to go into a completely different file and change numbers for it to work (Associated DB Tables Upon Character Creation - Third Party Support - rAthena).
-
The solution: go into rAthena folder -> rathena -> conf -> battle -> skill.conf . Look for "skill_min_damage" and set the answer to "0" (zero). What is happening is that if you have this turned on, it changes how the damage is calculated, so everything does at least 1 damage. It was meant to make it so you do more damage to plants, but it messes up the ability for a person to heal from damage. I have my heal back already, though I don't have my client set yet to show green numbers from heal damage, so it just looks like a white powder hitting me when the enemy hits. If you set your resistance to something like high, like 200+, you will see your hp bar jump back up when hit with that element. Note: I know this topic is older, but I guarantee others have the same problem, and it's incredibly frustrating. There is also no answer anywhere else on the boards.
-
Except if you are playing renewal, you will want to go into your renewal folder (re) instead of the pre-re one suggested above. The file you are looking for is the attr_fix.txt one. (Folder path: rAthena->rathena->db->re->attr_fix)
-
So I didn't find the perfect way to do it, I'm sure, but I found A way to do it. I went into the "battle_damage" function in battle.cpp, and I added: if (attack_type&BF_NORMAL) status_fix_spdamage(src, src, status_get_max_sp(src)/100, 0); Meaning for both players and mobs (and likely others), it zaps 1% sp per normal attack. Haven't tested it with other mercs, pets, homs, or etc, but it seems to be working so far. It still allows people to keep attacking with 0 sp, but I fixed that by lowering the damage to 5% of what it normally would be, making into an "I'm exhausted, and all I can do is weakly swing my arms about" struggle feature. It works quite nicely.
-
How do I get normal attacks to use SP? I want to do this for every enemy and player type I can. Adding "status_zap" in various places hasn't worked for me yet, but perhaps I'm doing it in the wrong places.
-
I'm looking to add regen for mobs. I think I've changed everything I need to except for one piece: * Gets the regeneration info of the given bl * @param bl: Object whose regen info to get [PC|HOM|MER|ELEM] //mob added AP * @return regen data or NULL if any other bl->type than noted above */ struct regen_data *status_get_regen_data(struct block_list *bl) { nullpo_retr(NULL, bl); switch (bl->type) { case BL_PC: return &((TBL_PC*)bl)->regen; case BL_HOM: return &((TBL_HOM*)bl)->regen; case BL_MER: return &((TBL_MER*)bl)->regen; case BL_ELEM: return &((TBL_ELEM*)bl)->regen; case BL_MOB: return &((TBL_MOB*)bl)->regen; default: return NULL; } } The compiler is saying that regen is not a member/part of TBL_MOB. How do I add regen to TBL_MOB? Anyone know? I'm not familiar with C++, so my weak attempts have failed. (The above code is found in status.cpp.)
-
I've loved digging into video game design by working on RO stuff. Right now, I'm working on balanced enemies. I'm looking for a formula that will allow me to increase an enemy's power compared to player level. I originally thought it would be a simple "boost all stats by 50% and it's a 50% power boost over the player," but that's not true mathematically. If I boost HP by 50% and I boost attack by 50%, the enemy now has 125% boost over the player ( 1.5 x 1.5 = 2.25 (minus 1 from 100% base power)). Anyone know a formula that would allow me to take a number of stats and apply the same boost to each of them so that if I wanted a 10% overall power boost against the player it would work (or a 50% boost or a 100% boost)? I'm doing this likely for monsters as they level, but I also plan to make different ranks of monsters- minion, lieutenant, boss type of stuff- so there is more variety on each map. I imagine fractions would be the easiest to use, because I've found using fractions has helped immensely in powering balancing so far for me.
-
I just found out RO made a poring that looks like a head of garlic. It's called Garling, and you can see it on this page (Pet System - NovaRO: Wiki (novaragnarok.com)). I didn't think they would stoop that low, but I guess they have. Perhaps I should make that the base poring for Lasagna. >.>
-
While surfing the web, I found this website that mentions some stuff that got dropped from RO during its development or later as it was running. I found it interesting. It also has a single picture of Zombie Dragon. Was not expecting Zombie Dragon to be a 3D boss. Link: Ragnarok Online - The Cutting Room Floor (tcrf.net)
-
While it isn't perfect, I finally figured how to game the aspd issue I was having. For the life of me, I couldn't find where that "50" was in the (50 / (200-aspd)) in the coding. BUT, the biggest thing that was important to me was wanting agility to have a clearer impact. I adjusted "temp_aspd", and along with some other small modifications- like setting one-handed weapons to leave a user with a base of 100 aspd when used- I was able to make it so that every 100 agility increased dps by 100% of my starting point. Meaning if I do 100 dps to start, it will allow me to do 200 dps with 100 agility, and then 300 dps with 200 agi, and so on. I'm super pumped about this, because now it will balance strength when I'm finished, and I have some clearer calculations I can work with. I hated all the "aspd corrections" and "we'll throw in some extra fractions for the fun of it." Just give me straight numbers! I also figured how to adjust my two-handed weapons to hit half as often (time-wise- 1 hit every 4 seconds vs 1 hit every 2 seconds), while still having my aspd mod make it so the dps speed boost from agi stayed the same. Since my two-handed weapons hit twice as hard, it finally adds a truly new playstyle to the game. Instead of the wishy-washy "this dagger does more damage than that mace" of standard RO play, I can finally have options- heavy damage user, normal damage user, tankier low damage user, etc. *Breathes in deeply* Ahhhh. I look forward to working out the rest of the kinks so playing different characters feels a lot more fresh and alive again. I can't wait to finally turn my crossbows into one-handed bows, and run around as a tanky trapper archer with low damage.
-
Have you tried giving your monsters skills that change their weapon element? I know the taekwon class has a move that can do that, and there is an itemskill move that I believe has all the elements (The itemskill is called "ITEM_ENCHANTARMS", and if you can figure out what number that skill is, you could possibly add to a mob's skill list with a high % chance to use it). Outside of that, I did a dirty source mod that made it so that my monsters attack with neutral element 50% of the time and their defensive element 50% of the time. My code means that a dark monster would be using dark basic attacks 50% of the time.