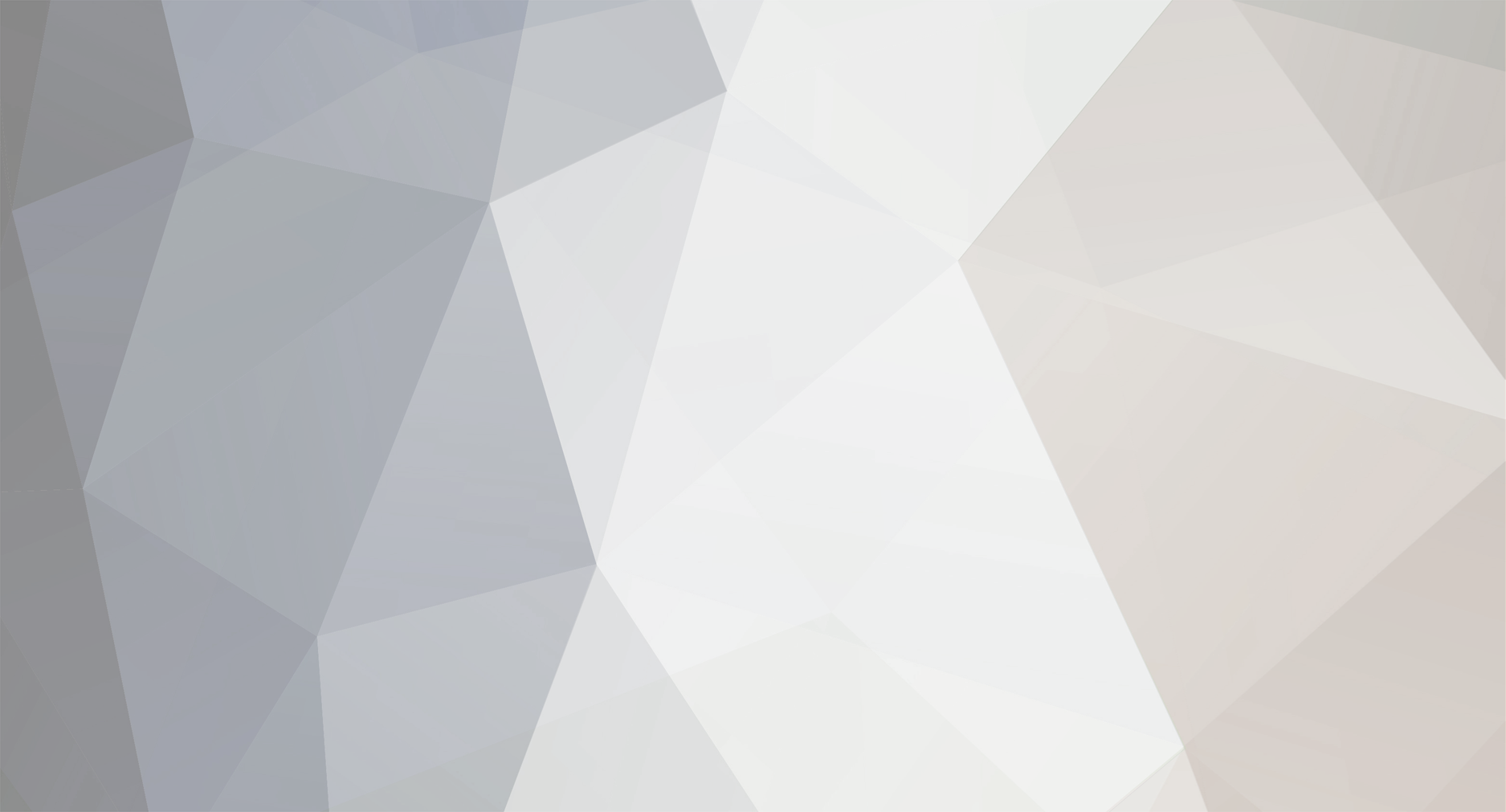
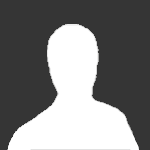
drupi
-
Posts
6 -
Joined
-
Last visited
Content Type
Profiles
Forums
Downloads
Jobs Available
Server Database
Third-Party Services
Top Guides
Store
Crowdfunding
Posts posted by drupi
-
-
instead of
getitem <itemID>,<qty>;
use
rentitem <itemID>,<duration>;
note that duration is in seconds
-
1
-
-
3 hours ago, Haziel said:
Short answer: yes.
Long answer: get player variables:
Zeny - Amount of Zeny. Hp - Current amount of hit points. MaxHp - Maximum amount of hit points. Sp - Current spell points. MaxSp - Maximum amount of spell points. StatusPoint - Amount of status points remaining. SkillPoint - Amount of skill points remaining. BaseLevel - Character's base level. JobLevel - Character's job level. BaseExp - Amount of base experience points. JobExp - Amount of job experience points. NextBaseExp - Amount of base experience points needed to reach the next level. NextJobExp - Amount of job experience points needed to reach the next level. Weight - Amount of weight the character currently carries. MaxWeight - Maximum weight the character can carry. Sex - 0 if female, 1 if male. Class - Character's job. Upper - 0 if the character is a normal class, 1 if advanced, 2 if baby. BaseClass - The character's 1-1 'normal' job, regardless of Upper value. For example, this will return Job_Acolyte for Acolyte, Priest/Monk, High Priest/Champion, and Arch Bishop/Sura. If the character has not reached a 1-1 class, it will return Job_Novice. BaseJob - The character's 'normal' job, regardless of Upper value. For example, this will return Job_Acolyte for Acolyte, Baby Acolyte, and High Acolyte. Karma - The character's karma. Karma system is not fully functional, but this doesn't mean this doesn't work at all. Not tested. Manner - The character's manner rating. Becomes negative if the player utters words forbidden through the use of 'manner.txt' client-side file.
Check player level, calculate the amount of exp for next level, calculate how many points is 25%, give experience.
bonusEXP = (BaseExp+NextBaseExp)*.25;
BaseExp=BaseEXP+bonusEXP;
*not the actual code but should give you the idea on how to implement this.
-
1
-
-
have you tried putting checkpoints on your script?
*pseudocode*
script{ #checkpoint =0; if (checkpoint==0) { //do something //since this part of the script is done, checkpoint+=; } if(checkpoint==1) { //do something //since this part of the script is done, checkpoint+=; } }
this way, if your server is done with a part of the script, after you reset your server, the script will resume to the last part it has completed.
-
//===== rAthena Script ======================================= //= Job Master //===== By: ================================================== //= Euphy //===== Current Version: ===================================== //= 1.3 //===== Compatible With: ===================================== //= rAthena SVN r16114+ //===== Description: ========================================= //= A fully functional job changer. //===== Additional Comments: ================================= //= 1.1 Fixed reset on Baby job change. [Euphy] //= 1.2 Added Expanded Super Novice support and initial Kagerou/Oboro support. [Euphy] //= 1.3 Kagerou/Oboro added. [Euphy] //============================================================ payon,166,97,6 script Job Master 113,{ function Job_Menu; function A_An; mes "[Job Master]"; if (Class > 4049) { mes "No more jobs are available."; close; } if (checkfalcon() || checkcart() || checkriding() || ismounting()) { mes "Please remove your "+((checkfalcon())?"falcon":"")+((checkcart())?"cart":"")+((checkriding())?"Peco":"")+((ismounting())?"mount":"")+" before proceeding."; close; } if (.SkillPointCheck && SkillPoint) { mes "Please use all your skill points before proceeding."; close; } set .@eac, eaclass(); set .@i, ((.ThirdClass)?roclass(.@eac&EAJ_UPPERMASK):Class); if (.@i > 6 && .@i < 22) { if (BaseLevel < .Rebirth[0] || JobLevel < .Rebirth[1]) { set .@blvl, .Rebirth[0]-BaseLevel; set .@jlvl, .Rebirth[1]-JobLevel; mes "You need "+((.@blvl>0)?.@blvl+" more base levels "+((.@jlvl>0)?"/ ":""):"")+((.@jlvl>0)?.@jlvl+" more job levels ":"")+"to continue."; close; } if (Class > 21) { mes "Switch to third class?"; next; Job_Menu(roclass(.@eac|EAJL_THIRD)); close; } while(1) { mes "Select an option."; next; set .@i, select(" ~ ^0055FFRebirth^000000: ~ ^777777Cancel^000000"); if (.@i==3) close; mes "[Job Master]"; mes "Are you sure?"; next; Job_Menu(((.@i==1)?4001:roclass(.@eac|EAJL_THIRD))); mes "[Job Master]"; } } set .@j1, roclass(.@eac|EAJL_2_1); set .@j2,roclass(.@eac|EAJL_2_2); if ((.@eac&EAJ_UPPERMASK) == EAJ_SUPER_NOVICE) setarray .@exp[0],roclass(.@eac|EAJL_THIRD),99; if (Class == Job_Ninja) setarray .@exp[0],.@j1,70; if (.@exp[0] && .ThirdClass) { if (BaseLevel < .Rebirth[0] || JobLevel < .@exp[1]) { set .@blvl, .Rebirth[0]-BaseLevel; set .@jlvl, .@exp[1]-JobLevel; mes "You need "+((.@blvl>0)?.@blvl+" more base levels "+((.@jlvl>0)?"/ ":""):"")+((.@jlvl>0)?.@jlvl+" more job levels ":"")+"to continue."; close; } mes "Switch to "+jobname(.@exp[0])+"?"; next; Job_Menu(.@exp[0]); close; } if (.@eac&EAJL_2) if (.@eac&(EAJL_UPPER|EAJL_BABY) || roclass(.@eac|EAJL_UPPER) == -1) { mes "No more jobs are available."; close; } if ((.@eac&EAJ_BASEMASK) == EAJ_NOVICE) { if (JobLevel < .JobReq[0]) mes "A job level of "+.JobReq[0]+" is required to change into the 1st Class."; else if (Class == 4001 && .LastJob && lastJob) { mes "Switch classes now?"; next; Job_Menu(roclass((eaclass(lastJob)&EAJ_BASEMASK)|EAJL_UPPER)); } else switch(Class) { case 0: Job_Menu(1,2,3,4,5,6,23,4046,24,25,4023); case 4001: Job_Menu(4002,4003,4004,4005,4006,4007); case 4023: Job_Menu(4024,4025,4026,4027,4028,4029,4045); default: mes "An error has occurred."; break; } close; } if (roclass(.@eac|EAJL_2_1) == -1 || roclass(.@eac|EAJL_2_2) == -1) mes "No more jobs are available."; else if (!(.@eac&EAJL_2) && JobLevel < .JobReq[1]) mes "A job level of "+.JobReq[1]+" is required to change into the 2nd Class."; else if (.LastJob && lastJob && (.@eac&EAJL_UPPER)) { mes "Switch classes now?"; next; Job_Menu(lastJob+4001); } else Job_Menu(.@j1,.@j2); close; function Job_Menu { while(1) { if (getargcount() > 1) { mes "Select a job."; set .@menu$,""; for(set .@i,0; .@i<getargcount(); set .@i,.@i+1) set .@menu$, .@menu$+" ~ "+jobname(getarg(.@i))+":"; set .@menu$, .@menu$+" ~ ^777777Cancel^000000"; next; set .@i, getarg(select(.@menu$)-1,0); if (!.@i) close; if ((.@i == 23 || .@i == 4045) && BaseLevel < .SNovice) { mes "[Job Master]"; mes "A base level of "+.SNovice+" is required to turn into a "+jobname(.@i)+"."; close; } mes "[Job Master]"; mes "Are you sure?"; next; } else set .@i, getarg(0); if (select(" ~ Change into ^0055FF"+jobname(.@i)+"^000000 class: ~ ^777777"+((getargcount() > 1)?"Go back":"Cancel")+"^000000")==1) { mes "[Job Master]"; mes "You are now "+A_An(jobname(.@i))+"!"; if (.@i==4001 && .LastJob) set lastJob, Class; jobchange .@i; if (.@i==4001 || .@i==4023) resetlvl(1); //set StatusPoint,StatusPoint + 4891; specialeffect2 338; specialeffect2 432; if (.Platinum) callsub Get_Platinum; close; } if (getargcount() == 1) return; mes "[Job Master]"; } end; } function A_An { setarray .@A$[0],"a","e","i","o","u"; set .@B$, "_"+getarg(0); for(set .@i,0; .@i<5; set .@i,.@i+1) if (compare(.@B$,"_"+.@A$[.@i])) return "an "+getarg(0); return "a "+getarg(0); } Get_Platinum: skill 142,1,0; switch(BaseClass) { case 0: if (Class !=23) skill 143,1,0; break; case 1: skill 144,1,0; skill 145,1,0; skill 146,1,0; break; case 2: skill 157,1,0; break; case 3: skill 147,1,0; skill 148,1,0; break; case 4: skill 156,1,0; break; case 5: skill 153,1,0; skill 154,1,0; skill 155,1,0; break; case 6: skill 149,1,0; skill 150,1,0; skill 151,1,0; skill 152,1,0; break; default: break; } switch(roclass(eaclass()&EAJ_UPPERMASK)) { case 7: skill 1001,1,0; break; case 8: skill 1014,1,0; break; case 9: skill 1006,1,0; break; case 10: skill 1012,1,0; skill 1013,1,0; break; case 11: skill 1009,1,0; break; case 12: skill 1003,1,0; skill 1004,1,0; break; case 14: skill 1002,1,0; break; case 15: skill 1015,1,0; skill 1016,1,0; break; case 16: skill 1007,1,0; skill 1008,1,0; skill 1017,1,0; skill 1018,1,0; skill 1019,1,0; break; case 17: skill 1005,1,0; break; case 18: skill 238,1,0; break; case 19: skill 1010,1,0; break; case 20: skill 1011,1,0; break; default: break; } return; OnInit: setarray .Rebirth[0],99,50; // Minimum base level, job level to rebirth OR change to third class setarray .JobReq[0],10,40; // Minimum job level to turn into 1st class, 2nd class set .ThirdClass,0; // Enable third classes? (1: yes / 0: no) set .SNovice,45; // Minimum base level to turn into Super Novice set .LastJob,1; // Enforce linear class changes? (1: yes / 0: no) set .SkillPointCheck,0; // Force player to use up all skill points? (1: yes / 0: no) set .Platinum,1; // Get platinum skills automatically? (1: yes / 0: no) end; }
Here's a working job changer. Credits to Euphy in making this script
-
Hi.
I'm thinking of creating an event that simulates what happens on Thanatos Tower but modified in a way that the entire server should participate in order to clear it. Of course, if cleared, everyone who participated will receive a form of reward
1. is this a good idea?
if yes, 2. has this been done before?
if no, 3. where do we start?
[DISCONTINUED] Secret's Updated NEMO Patches
in Client Releases
Posted · Edited by drupi
Hi. Im trying to use the "disable multiple windows". it says patch complete but i can still run multiple windows. please advice. I'm using 2015-11-04
nvm. fixed :)) i was just being stupid