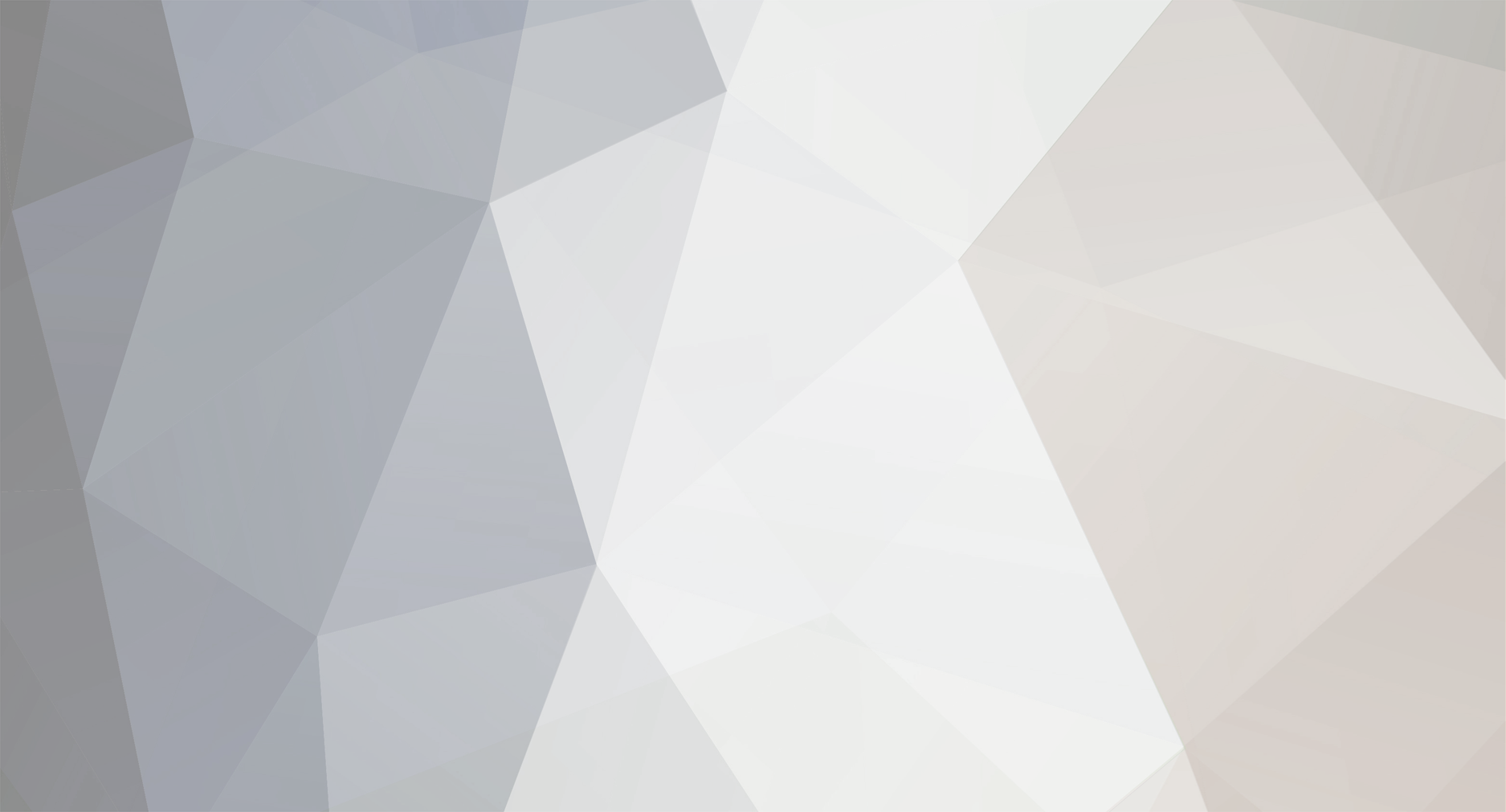
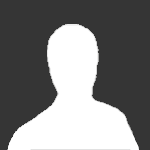
GubA
-
Posts
24 -
Joined
-
Last visited
Content Type
Profiles
Forums
Downloads
Jobs Available
Server Database
Third-Party Services
Top Guides
Store
Crowdfunding
Posts posted by GubA
-
-
-
How to edit FluxCP to write thai language.
When i write thai it will alien language.Thank you for help
-
-
-
3 hours ago, Emistry said:
it shall be safe to ignore these kind of warning up to current version of MySQL 8.0+
If you'd like to avoid these warnings and play safe, update all your affected tables having column type definitions of something like INT(##) to INT (i.e. without explicitly specifying the display width)
Thank you.
But.
How to update all your affected tables.
I use AppServ 9.3.0.
-
When i import main.sql will show
Warning: #1681 Integer display width is deprecated and will be removed in a future release.How to fix this.
Thank you.
-
2 hours ago, Emistry said:
just disable the setting if you dont need the feature? what's the point of changing it to auto berserk?
i need only afk.
dont need autotrade.
-
On 1/17/2023 at 9:48 PM, Emistry said:
- script KillMon2Cash -1,{ OnNPCKillEvent: .@gettimetick = gettimetick(2); if (rand(100) < 1 && .@gettimetick > @delay) { getitem 50001,1; @delay = .@gettimetick + 60; // 60 seconds } end; }
Thank you too much.
-
This my code
QuoteACMD_FUNC(afk) {
nullpo_retr(-1, sd);if( map_getmapflag(sd->bl.m, MF_AUTOTRADE) != battle_config.autotrade_mapflag ) {
clif_displaymessage(fd, msg_txt(sd,1179)); // Autotrade is not allowed on this map.
return -1;
}if( pc_isdead(sd) ) {
clif_displaymessage(fd, msg_txt(sd,1180)); // You cannot autotrade when dead.
return -1;
}sd->state.autotrade = 1;
if( battle_config.at_timeout ) {
int timeout = atoi(message);
status_change_start(NULL,&sd->bl, SC_AUTOTRADE, 10000, 0, 0, 0, 0, ((timeout > 0) ? min(timeout,battle_config.at_timeout) : battle_config.at_timeout) * 60000, 0);
}
//clif_changelook(&sd->bl,LOOK_HEAD_TOP,471);
channel_pcquit(sd,0xF); //leave all chan
clif_authfail_fd(sd->fd, 15);return 0;
}Can i change SC_AUTOTRADE >>> SC_AUTOBERSERK
because when i recall it will hide.
Thank you for help.
-
Quote
- script KillMon2Cash -1,{
end;OnNPCKillEvent:
if(rand(1,100) < 1) getitem 50001,1;
end;
}Thank you to help.
-
What I want is
1. The player's level is checked first.
2. Set items for players to exchange for levels.example
If a player is level 1, must bring 5 Red Potions and 10 Fly Wings in exchange for leveling up 1.
When player level 2 must exchange 10 bottles of Awakening Potion 20 Jellopy for level up 1etc.
Thank you for help. -
How to fix this map.
i has download KRO JRO IRO but cant go this map.SpoilerSpoilerSpoilerSpoilerThank for Help.
Thank you.
-
8 minutes ago, Radian said:
I think in these list. you need to double check all the items that has been added, one or two might not be in the database that's why its saying non-existent error message.
setarray .rare_ID0,5909; setarray .rare_ID1,5912; setarray .rare_ID2,5914; setarray .rare_ID3,5915; setarray .rare_ID4,5977; setarray .rare_ID5,5979; setarray .rare_ID6,5980; setarray .rare_ID7,15280; setarray .rare_ID8,15841; setarray .rare_ID9,15843; setarray .rare_ID10,15858;
all item have in db i has check
-
prontera,155,177,5 script random 615,{ function Getcostume; if( countitem( .rare_ticket ) ) { mes "Rare Ticket"; .@r = .rate_chance_rare; // chance .@ticket = .rare_ticket ; } else if( countitem( .normal_ticket ) ) { mes "Normal Ticket"; .@r = .rate_chance_normal; // chance .@ticket = .normal_ticket; } else { mes "random"; close; } if( select( "go", "no" ) -1 ) close; delitem .@ticket, 1; if( .@r > rand( 1, 1000 ) ) { // chance 50% for rare ticket mes "random"; getitem Getcostume( "rare" ), 1; close; } else { mes "random"; getitem Getcostume( "normal" ), 1; close; } OnInit: .normal_ticket = 501; .rare_ticket = 502; .rate_chance_rare = 500; // chance to get a rare costume with rare ticket .rate_chance_normal = 100; // chance to get a rare costume with normal ticket // rare costume ID // size array must be < 128 // use the synthaxe .rare_IDX for adding news array // ------------------------------------------------ setarray .rare_ID0,5909; setarray .rare_ID1,5912; setarray .rare_ID2,5914; setarray .rare_ID3,5915; setarray .rare_ID4,5977; setarray .rare_ID5,5979; setarray .rare_ID6,5980; setarray .rare_ID7,15280; setarray .rare_ID8,15841; setarray .rare_ID9,15843; setarray .rare_ID10,15858; // normal costume ID // size array must be < 128 // use the synthaxe .normal_IDX for adding news array // ------------------------------------------------ setarray .normal_ID0,50001; setarray .normal_ID1,50001; setarray .normal_ID2,50001; setarray .normal_ID3,50001; setarray .normal_ID4,50001; setarray .normal_ID5,50001; setarray .normal_ID6,50001; setarray .normal_ID7,50001; // Don't touch // ----------- callsub L_size, "rare"; callsub L_size, "normal"; end; L_size: while( getd( "."+ getarg(0) +"_ID"+ .@i +"[0]" ) ) { setd ".size_"+ getarg(0) +""+ .@i, getarraysize( getd( "."+ getarg(0) +"_ID"+ .@i ) ); setd ".size_tot_"+ getarg(0), getd( ".size_tot_"+ getarg(0) ) + getarraysize( getd( "."+ getarg(0) +"_ID"+ .@i ) ); .@i++; } return; function Getcostume { .@r = rand( 1, getd( ".size_tot_"+ getarg(0) ) ); .@i = -1; while( .@r > .@tmp ) .@tmp = .@tmp + getd( ".size_"+ getarg(0) + .@i++ ); .@in = .@r - ( .@tmp - getd( ".size_"+ getarg(0) + .@i ) ) -1; return getd( "."+ getarg(0) +"_ID"+ .@i +"[ "+ .@in +" ]" ); } }
-
i use data KRO and GitHub - llchrisll/ROenglishRE: An unofficial english translation project for Korea Ragnarok Online (kRO).
my DATA.ini
0=server.grf
1=data.grf
but don't have it
[20931] = {
unidentifiedDisplayName = "Prism Rangers Scarf [0]",
unidentifiedResourceName = "Rainbow_Scarf_Jp",
unidentifiedDescriptionName = {
""
},
identifiedDisplayName = "Prism Rangers Scarf",
identifiedResourceName = "Rainbow_Scarf_Jp",
identifiedDescriptionName = { -
i see this src
and i want to add this command
how to add this in my rathena
-
Thank you every body i can fix this
-
This my sclientinfo/clientinfo
<?xml version="1.0" encoding="euc-kr" ?> <clientinfo> <desc>ragnarok</desc> <servicetype>korea</servicetype> <servertype>primary</servertype> <connection> <display>ragnarok</display> <address>127.0.0.1</address> <port>6900</port> <version>55</version> <langtype>5</langtype> <registrationweb>www.ragnarok.com</registrationweb> <loading> <image>loading00.jpg</image> <image>loading01.jpg</image> <image>loading02.jpg</image> <image>loading03.jpg</image> <image>loading04.jpg</image> <image>loading05.jpg</image> <image>loading06.jpg</image> </loading> <aid> <admin>2000000</admin> </aid> </connection> </clientinfo>
My exe 2021-01-07aRagexeRE and diff like this
8 Custom Window Title 9 Disable 1rag1 type parameters (Recommended) 13 Disable Ragexe Filename Check (Recommended) 14 Disable Hallucination Wavy Screen (Recommended) 23 Enable /who command (Recommended) 24 Fix Camera Angles (Recommended) 34 Enable /showname (Recommended) 36 Always read msgstringtable.txt (Recommended) 38 Remove Gravity Ads (Recommended) 39 Remove Gravity Logo (Recommended) 41 Disable Nagle Algorithm (Recommended) 44 Translate Client (Recommended) 46 Use Normal Guild Brackets (Recommended) 47 Use Ragnarok Icon 48 Use Plain Text Descriptions (Recommended) 49 Enable Multiple GRFs (Recommended) 53 Use Ascii on All LangTypes (Recommended) 64 @ Bug Fix (Recommended) 65 Load Custom lua file instead of iteminfo*.lub (Recommended) 73 Remove Hourly Announce (Recommended) 84 Remove Serial Display (Recommended) 90 Enable DNS Support (Recommended) 213 Disable Help Message on Login (Recommended) 230 Always load Korea ExternalSettings lua file (Recommended) 231 Remove hardcoded address/port (Recommended) 232 Restore old login packet (Recommended) 270 Change AchievementList*.lub path 271 Change MonsterSizeEffect*.lub path 272 Change Towninfo*.lub path 273 Change PetEvolutionCln*.lub path 274 Change Tipbox*.lub path 275 Change CheckAttendance*.lub path 276 Change OngoingQuestInfoList*.lub path 277 Change RecommendedQuestInfoList*.lub path 278 Change PrivateAirplane*.lub path 290 Hide build info in client (Recommended) 291 Hide packets from peek (Recommended) 313 Change MapInfo*.lub path 326 Disable OTP Login Packet (Recommended) 338 Additional client validation (Recommended) 342 Add support for preview button in cash shop 348 Send client flags to server (Recommended) 362 Fixes the Korean Job name issue with LangType (Recommended) 404 Use borderless mode in full screen (Recommended) 427 Check is servertype in xml file was set to correct value (Recommended) 438 Set fixed server ip address 449 Add support for preview button in custom shops
-
On 12/19/2022 at 1:26 AM, Singe Horizontal said:
Which script language is this
when write script , what language i must use?
On 12/19/2022 at 7:14 AM, GubA said:when write script , what language i must use?
Sorry i don't know.
i see other script and write think is right.
On 12/17/2022 at 4:27 PM, lelouchxv said:show the error at your console so anyone here can help you much better.
This mt error
-
// This script causes monsters to drop a red potion when they are killed. function onMonsterDeath(monster, killer) { // Check if the killer is a player. if (killer.isPlayer()) { // Drop a red potion. monster.dropItem(501, 1); } } module.exports = { onMonsterDeath: onMonsterDeath };
Thank you to help me
-
Sorry.
I'm Thai.
I not smart.
But i can read a little.
I want Data+Exe very much.
I download rAthena from this https://github.com/rathena/rathena
But I can't find data+exe for play.
Plese give for me.
Thank you very much.
Why error when compile
in Source Support
Posted
Original Code Is OK
static int buildin_autoattack_sub(struct block_list *bl,va_list ap)
{
int *target_id=va_arg(ap,int *);
*target_id = bl->id;
return 1;
}
void autoattack_motion(struct map_session_data* sd)
{
int i, target_id;
if( pc_isdead(sd) || !sd->state.autoattack ) return;
for(i=0;i<=9;i++)
{
target_id=0;
map_foreachinarea(buildin_autoattack_sub, sd->bl.m, sd->bl.x-i, sd->bl.y-i, sd->bl.x+i, sd->bl.y+i, BL_MOB, &target_id);
if(target_id){
unit_attack(&sd->bl,target_id,1);
break;
}
target_id=0;
}
if(!target_id && !pc_isdead(sd) && sd->state.autoattack){
unit_walktoxy(&sd->bl,sd->bl.x+(rand()%2==0?-1:1)*(rand()%25),sd->bl.y+(rand()%2==0?-1:1)*(rand()%25),0);
}
return;
}
But when edit like this
static int buildin_autoattack_sub(struct block_list *bl, va_list ap)
{
int *target_id = va_arg(ap, int *);
*target_id = bl->id;
return 1;
}
void autoattack_motion(struct map_session_data* sd)
{
static int last_attack_tick = -10000; // Initialize to a value below the current tick
int i, target_id, tick = gettick();
if (pc_isdead(sd) || !sd->state.autoattack) return;
if (tick - last_attack_tick >= 10000) { // 10 seconds since last attack
// Use Fly Wing
if (sd->status.inventory[0].nameid == 601) {
clif_useitem(sd, 0);
return;
}
} else {
// Search for target to attack
for (i = 0; i <= 9; i++) {
target_id = 0;
map_foreachinarea(buildin_autoattack_sub, sd->bl.m, sd->bl.x - i, sd->bl.y - i, sd->bl.x + i, sd->bl.y + i, BL_MOB, &target_id);
if (target_id) {
unit_attack(&sd->bl, target_id, 1);
last_attack_tick = tick;
break;
}
target_id = 0;
}
}
if (!target_id && !pc_isdead(sd) && sd->state.autoattack) {
unit_walktoxy(&sd->bl, sd->bl.x + (rand() % 2 == 0 ? -1 : 1) * (rand() % 25),
sd->bl.y + (rand() % 2 == 0 ? -1 : 1) * (rand() % 25), 0);
}
}
Is error.
How to fix this.
Thank you.